**Cubes Project Report**
**Heikki Ikäheimonen
heikki.ikaheimonen@gmail.com**
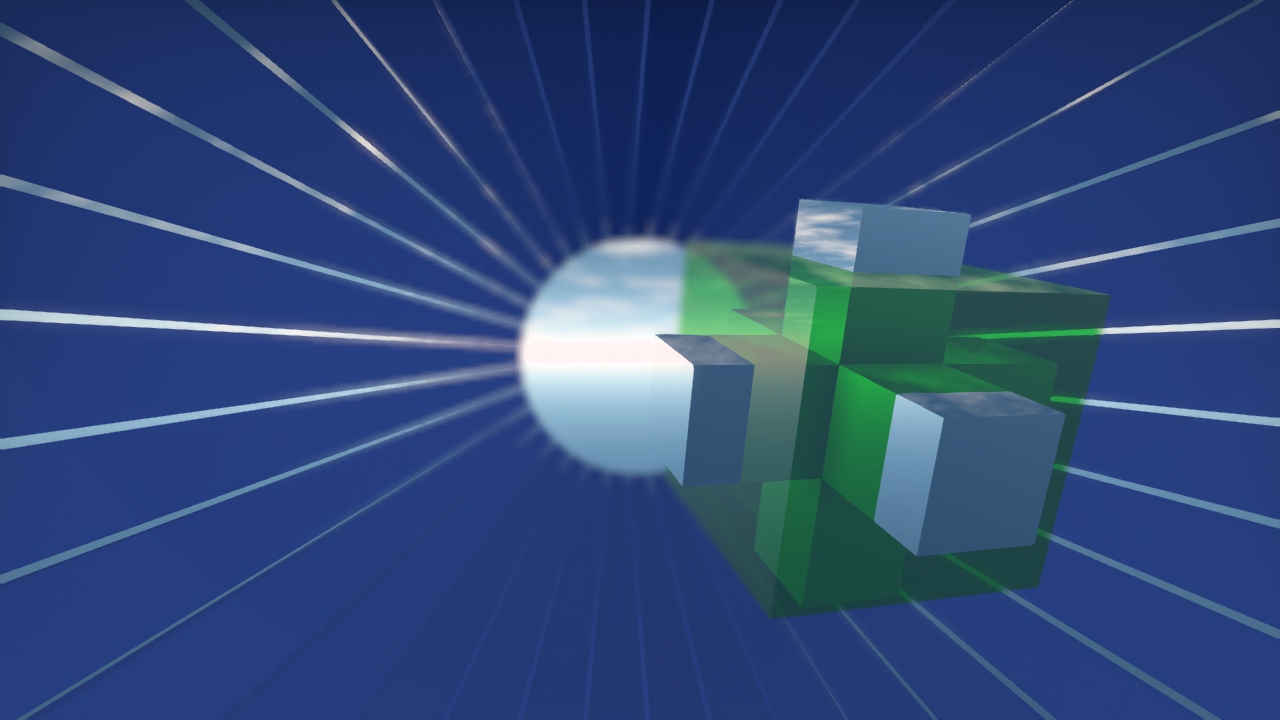
Introduction
=============================================================
This project introduced three different ways of creating scenes from simple cubes, by directly editing data file, by using GUI editor and by programmatically generating the scene file.
The project also had a warmup section to get familiar with the G3D engine and a questions section for testing understanding of the engine and aspects of programming.
The project specification can be found from http://graphicscodex.com/projects/cubes/index.html
System Overview
=============================================================
The cubes project is based on the starter project from G3D engines sample projects. The projects main entry point
is in the App class inheriting from GApp of the G3D engine. The project has only one other class, MySceneBuilder class that programmatically
creates two of the required scenes on program startup, the staircase scene and a scene of my own designing. Other scenes are in scene folder under data-file as a .Scene.Any files created by hand.
Major Class/Function | Description
---------------------|------------
[class App](../build/doc/class_app.html) | Initializes and runs the application and calls MySceneBuilder's build methods.
[class MySceneBuilder](../build/doc/class_my_scene_builder.html) | Builds and saves custom scenes to a scene file.
[MySceneBuilder::BuildStaircaseScene](../build/doc/class_my_scene_builder.html#a341d938f05fc1a32842c1c59c8520c09) | Builds the staircase scene file and saves to disk.
[MySceneBuilder::BuildCubeTunnelScene](../build/doc/class_my_scene_builder.html#add9df378fb23c2643ada169f063e1aaf) | BuildScene creates and saves my custom scene to disk.
Coordinate System
=====================================================
The default 3d coordinate system in G3D is right handed.
The following diagram describes x,y,z axes and the pitch, yaw and roll in the coordinate system.
*************************************************************************************************
* +y *
* ^ *
* | *
* | ◄╮ roll around z - axis *
* pitch around x-axis ⤹ +-----> +x *
* / ⤴ yaw around y -axis *
* v *
* +z *
*************************************************************************************************
Results
=====================================================
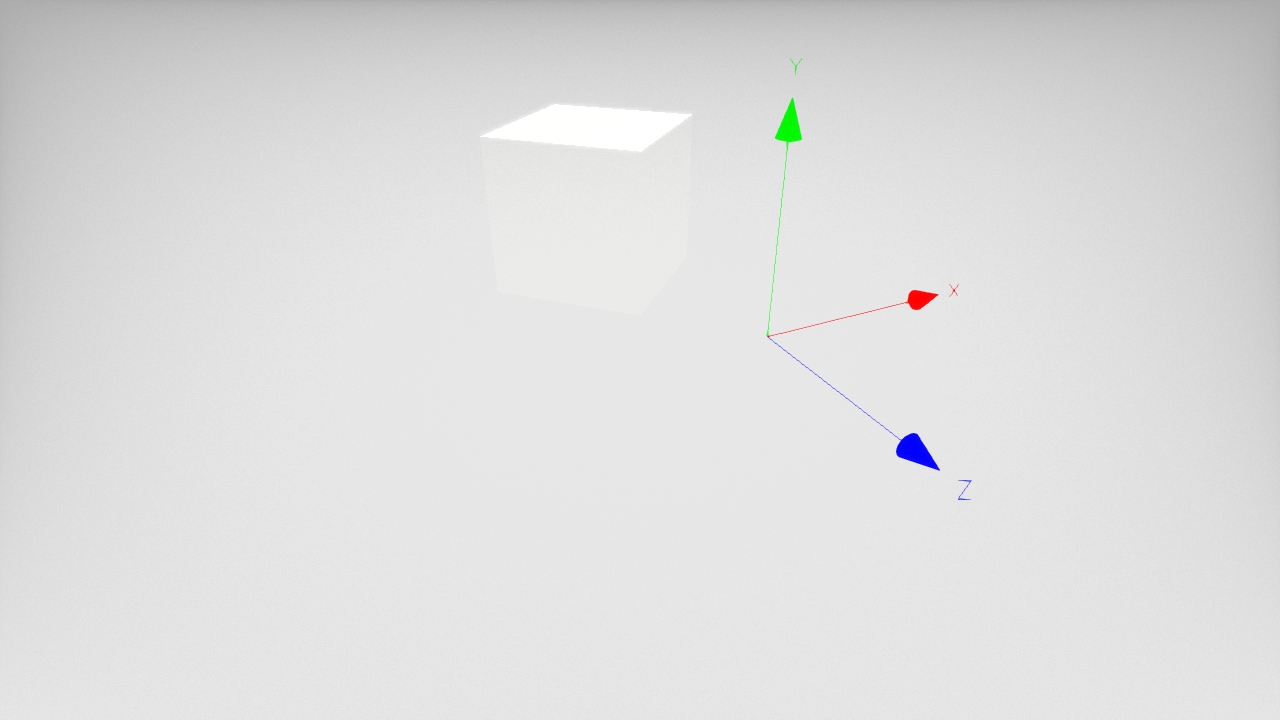
White cube scene showing one white cube rotated 45 degrees about vertical axis and translated -2 units on z-axis.
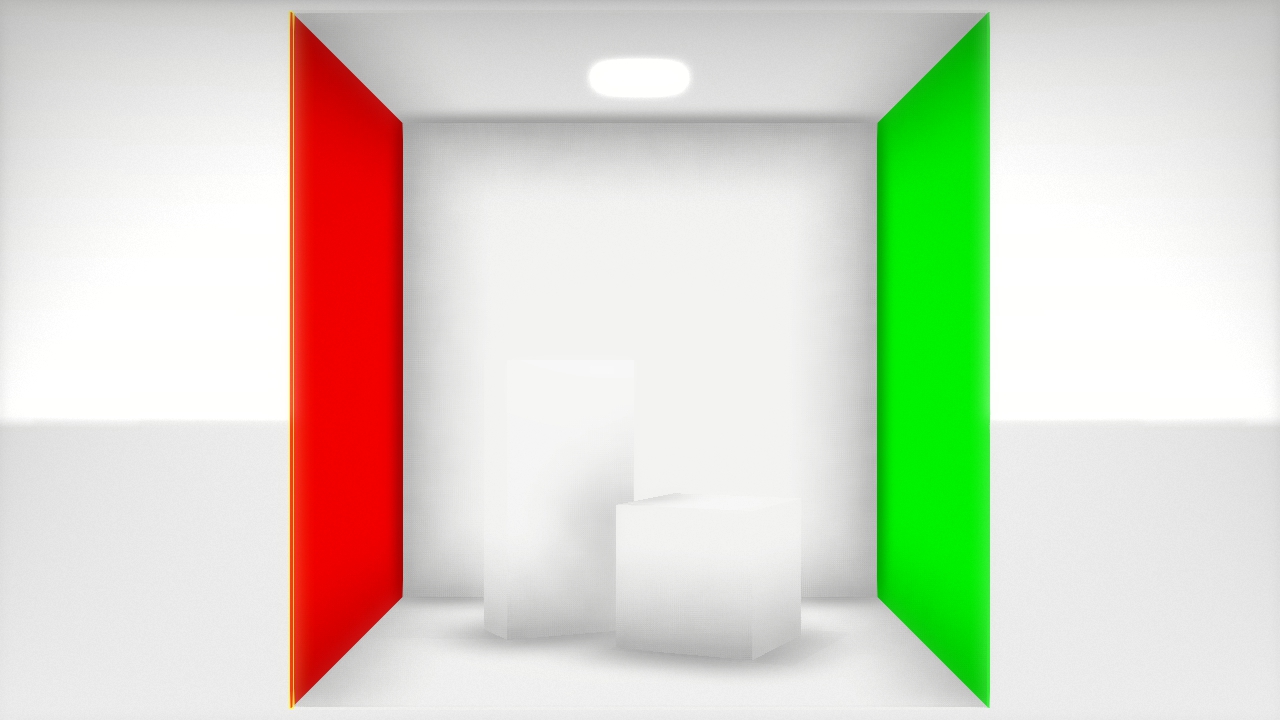
Cornell box scene where walls and boxes are tranformed crate.obj objects.
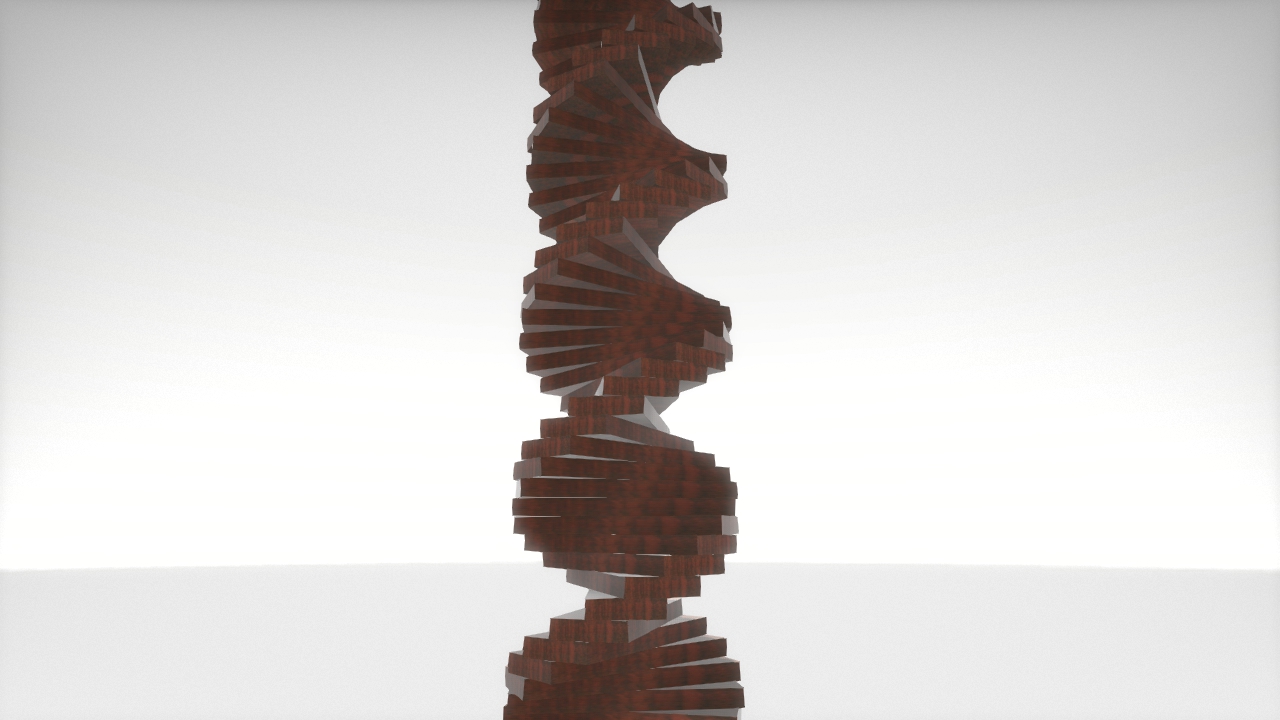
Scene that is created in C++ code from MySceneBuilder::BuildStaircaseScene creating a spiralling stairs consisting of 50 scaled and textured cubes.
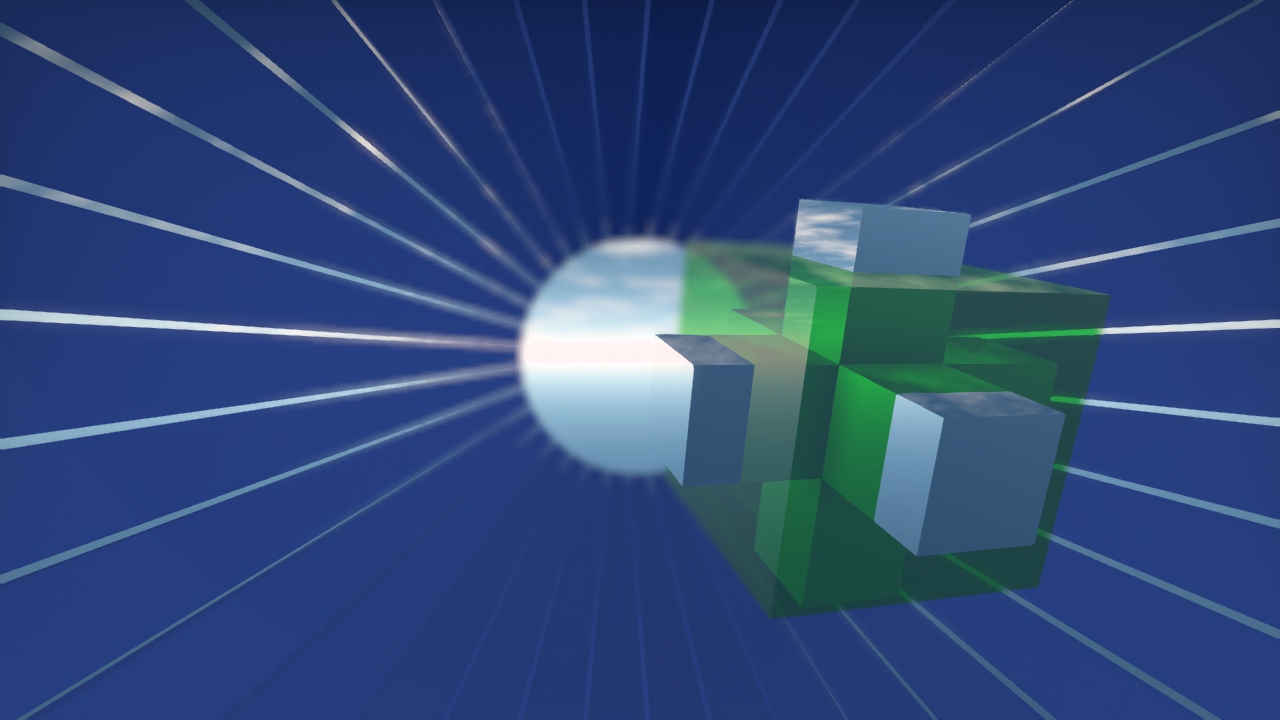
A Scene of my creation with depth of field blur giving it more artictic look.
Custom Scene Process
=====================================================
I wanted to make something abstract but simple and decided to make a tunnel made of cubes. I wanted to programmatically count the location of the cubes making the tunnel so I decided to create the scene in C++ by writing to std::ostream.
I decided to create 36 stretched cubes in for loop, I chose 36 so I can rotate each equally 10 degrees when counting the location of the cube, since I chose tunnel I could count the location as point on a circle in x-y plane and increasing angle by 10 degree every loop.
$$ x = r*cos(angle*i), y = r*sin(angle*i)$$ where r is range and i is the loop index from 0 to 35.
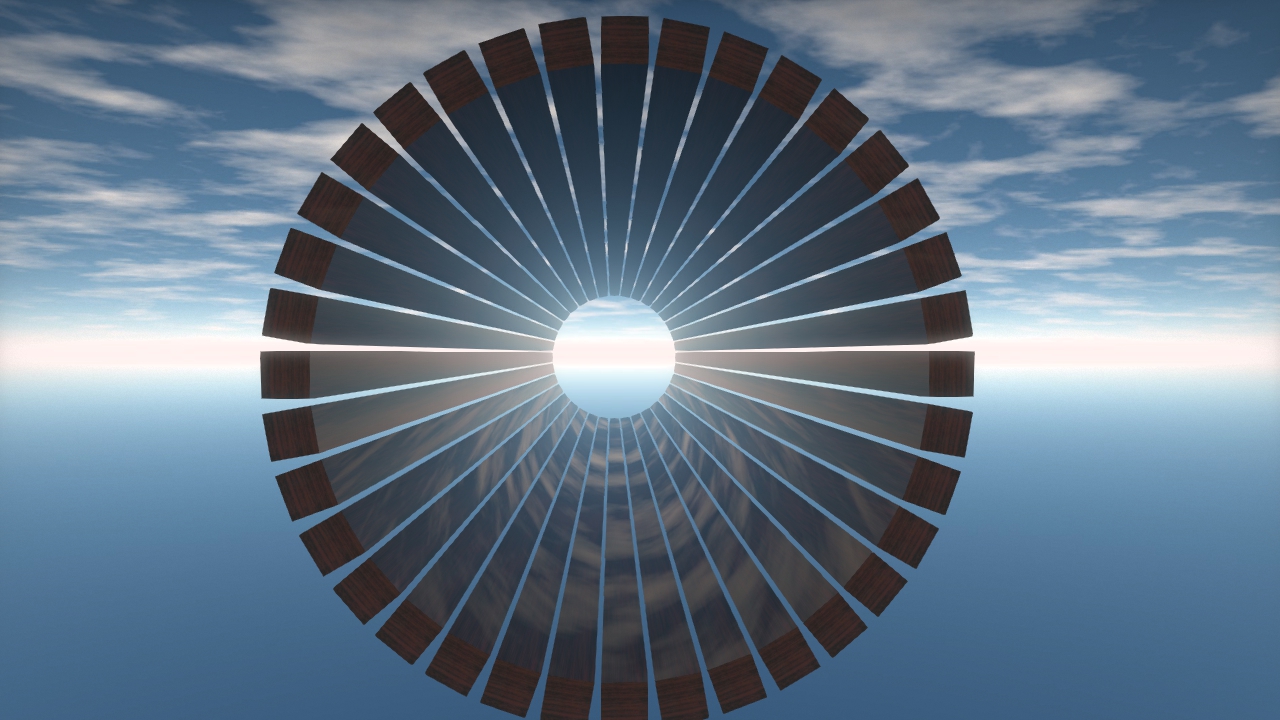
After this I wanted to add something to the middle of the tunnel.
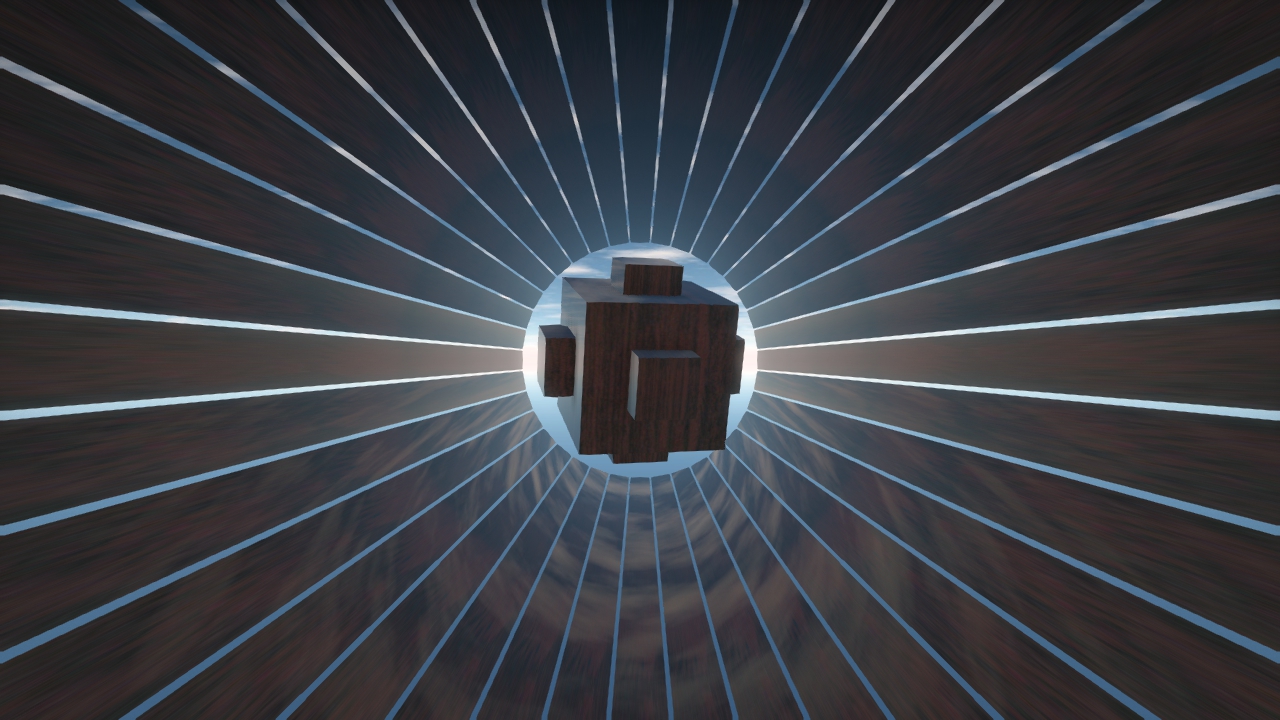
To make it look more interesting I started to experiment with materials. I also adjusted in the scene viewer the debug cameras settings for Depth of field to get more artistic look.
After I was happy with DoF settings and camera location I pressed save in the GUI and checked the modified file. From the modified file I copied by hand the camera settings to the C++ class.
The final scene had 40 cubes 36 making the tunnel and 4 making a cube object in middle, materials and also depth of field blur experimented from the debug camera gui.
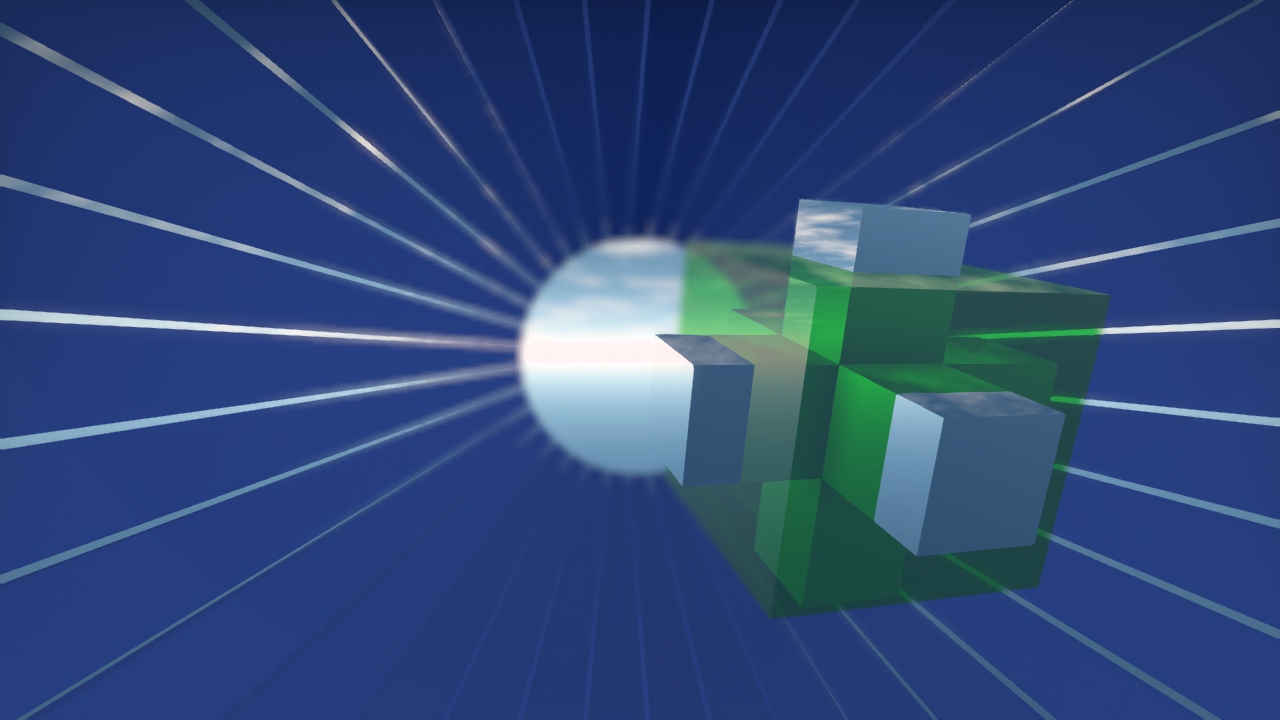
Typeset Equation in Markdeep
=====================================================
$$ \frac{df(x)}{dx} = \lim_{h\to 0}\left[\frac{f(x + h) - f(x -h)}{2h}\right]$$
Questions
=====================================================
1. What are the differences between the `Scene*` and `shared_ptr